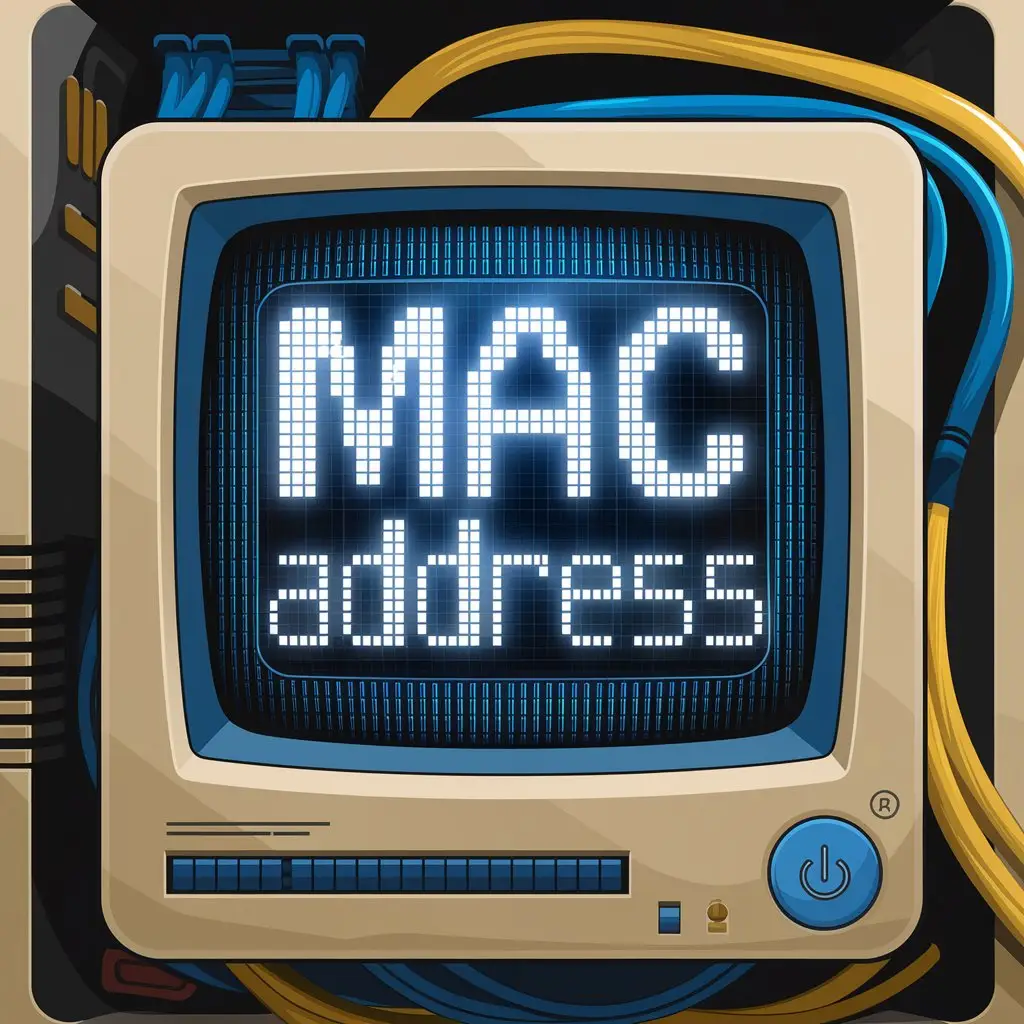
VCF Orchestrator is a powerful tool that helps administrators automate tasks in a VMware environment. One important task it can perform is finding the MAC address of virtual machines.
I once worked with a CMDB system that required manual updates each time a new VM was deployed. One of the necessary attributes was the MAC address, which is why I wrote this function.
That is a practical guide for getting the MAC address using the JavaScript function.
Some facts about MACs in vSphere
vSphere offers several methods for the automatic allocation of MAC addresses within the vCenter Server. The available schemes for MAC address generation include:
- VMware OUI allocation: This is the default method for allocating MAC addresses.
- Prefix-based allocation: This approach allows for the specification of a custom prefix when generating MAC addresses.
- Range-based allocation: This scheme allows for the allocation of MAC addresses from a specified range.
Once a MAC address is generated for a virtual machine, it remains unchanged unless a conflict arises with another registered virtual machine. It’s important to note that the MAC address of a powered-off virtual machine is not compared to the addresses of any running or suspended virtual machines. Consequently, when the virtual machine is powered back on, it may receive a different MAC address if there is a conflict with another virtual machine’s address.
Code
Let’s look at a generic function, which, preferably, should be used as an action element, how to get the MAC address from the VM.
function getVmMacAddress(vmObject) {
System.log('---------------------------------');
System.log('Get VM MAC Address');
var networkDeviceTypes = [
VcVirtualVmxnet3,
VcVirtualE1000,
VcVirtualPCNet32,
VcVirtualVmxnet,
VcVirtualVmxnet2,
];
var devices = vmObject.config.hardware.device;
var loop = function (device) {
if (networkDeviceTypes.some(function (type) { return device instanceof type; })) {
var macAddress = device.macAddress;
System.log("MAC Address: ".concat(macAddress));
return { value: macAddress };
}
};
for (var i = 0, device = devices; i < device.length; i++) {
var device = device[i];
var state = loop(device);
if (typeof state === "object")
return state.value;
}
System.log('No network device found');
return undefined;
};
Get MAC address function
Understanding the Code
Here’s a breakdown of the provided function:
- Logging: Logs the process start and any retrieved MAC addresses, which is helpful for troubleshooting.
- Device Types:
loop
functions checks for common VMware network adapter types (Vmxnet3, E1000, etc.) to identify the network device usingsome
.Some
returns boolean. It will returntrue
ifdevice
is an instance of one of thenetworkDeviceTypes
. If true, theloop
function will return a mac address of the NIC. - Device Loop: Iterates through all devices on the VM to find a valid network adapter and retrieve its MAC address.
Usage
Function call example. vm
is a VC:VirtualMachine
object.
var macAddress = getVmMacAddress(vm);
Use Cases
Those are common scenarios where retrieving a VM’s MAC address is beneficial include:
- Automated VM inventory management
- Network troubleshooting
- Security audits and compliance checks
💡
I also want to hear from you about how I can improve my topics! Please leave a comment with the following:
– Topics you’re interested in for future editions
– Your favorite part or take away from this one
I’ll do my best to read and respond to every single comment!